Overview
In Jetfire a 'workflow' refers to a workflow object. A Jetfire 'workflow' is an instantiation of a '
Workflow Class'. Jetfire workflow classes have a number of unique
first class attributes such as persistence,
dynamic access modifiers, and
states. A workflow object at its heart is still a standard
OO object that describes data and operations (workflow class) that operate on the data.
Jetfire objects are either workflow objects or
Intrinsic Object s.
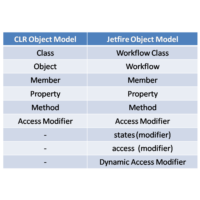
CLR vs Jetfire Object Model comparison
Unique features
In addition the Jetfire workflow objects have a number of feature not found in other languages.
- Workflow States
- Persistent
- Workflow objects are stored in a server nexus.
- Each workflow has a GUID supporting retrieval and tracking.
- Is automatically serialized to permanent storage.
- Upon start up workflow objects that reside in a fully cached workspace are automatically restored to client nexus.
- Workflow objects that are cached in 'partially' cached workspace are loaded on demand (when referenced) or when found as a result of a 'search' operation.
- Workflows can be made a 'Root Object' to prevent deletion by garbage collector.
- Integrated security
- Workflows can be assigned roles restricting which users have access to the workflow.
- Bound to source code
- Source code is compiled and dynamically linked to other source code.
- Code is represented as objects.
- Dynamic access modifiers
- Changes dynamically based on an optional 'access' or 'states' construct.
- The same workflow object can exist in multiple clients simultaneously.
- The server nexus is responsible for synchronizing updates.
- Workflow references can not be assigned 'null' unless declared 'nullable' (also see Void Safety).
Creating A Jetfire Workflow Object
A Jetfire object can be created programmatically by 2 techniques.
- Jetfire script using the 'new' operator.
- A workflow object can be created using the .net API.
The 'new' construct may optionally specify the following for the new workflow:
- be assigned the 'atroot' option to prevent garbage collection.
- be placed in a specific workspace.
Jetfire 'new' Operator
The new operator creates an instance of a workflow object. The new instance will be created in the same
workspace as the creating workflow object. If the new operator is executed by static code the new instance will be placed in the 'public' workspace by default.
namespace NewOperatorExample
{
public workflow Factory
{
// A method that creates a workflow object.
public MyFlow CreateMyFlow()
{
// the new operator creates a new instance
// of a MyFlow object;
return new MyFlow();
}
}
public workflow MyFlow
{
// Place methods, constructors and properties
// that define 'MyFlow' here.
}
}
Jetfire 'new' Operator 'root' Option
The '
root' option tells the 'Garbage Collector' not to collect the workflow even if the object has no references. This useful when container objects, such as
workspaces, are created programatically.
In order for the 'Garbage Collector' to collect 'root' objects they must be explicitly deleted.
namespace NewOperatorExample
{
public workflow Factory
{
// A method that creates a workflow object.
public workspace CreateWorkspace()
{
// the new operator creates a new instance
// of a workspace;
return new workspace() at root;
}
}
}
Creating a Workflow in a Specific Workspace
The 'in' option specifies which
workspace to place the workflow.
By default workflows are created in the same workspace as the creating workflow object. If the new operator is executed by static code the new instance will be placed in the 'public' workspace by default.
Specifying the workspace has no effect on the operation of workflow.
namespace NewOperatorExample
{
public workflow Factory
{
public static workspace MyCoolSpace
{
get;
private set;
}
// static constructor
Factory()
{
MyCoolSpace = new workspace("MyCoolSpace") at root;
}
// A method that creates a workflow object.
public Workflow CreateMyFlow()
{
// the new operator creates a new instance
// of a the class 'Flow' in the workspace 'MyCoolSpace'
return new Flow() in Factory.MyCoolSpace;
}
}
//this is a simple workflow that doesn't do much.
public workflow Flow
{
// code for the workflow should be place here
}
}
Assigning a Workflow Reference Null
A Workflow references can not be assigned null unless they are declared 'nullable'. By default uninitialized workflow references are assigned the
empty object. This is part of Jetfire's
void safety mechanisms.
namespace NullableExample
{
public workflow MyFlow
{
// references to MyClass are allowed to be assigned the null value.
MyClass myclass = null;
// 'myOtherObject' will be assigned 'MyClass.Empty()' since no initialization
// code was specified.
MyClass myOtherObject;
}
}
// reference the 'MyClass' can be assigned 'null'
public nullable workflow MyClass
{
// a do nothing workflow class
}
}
New Workflow Object using the Jetfire API
See Also